1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 392 393 394 395 396 397 398 399 400 401 402 403 404 405 406 407 408 409 410 411 412 413 414 415 416 417 418 419 420 421 422 423 424 425 426 427 428 429 430 431 432 433 434 435 436 437 438 439 440 441 442 443 444 445 446 447 448 449 450 451 452 453 454 455 456
#![doc = include_str!("../README.md")]
use geometry_rs::{Point, Polygon};
use std::collections::HashMap;
use std::f64::consts::PI;
use std::vec;
use tzf_rel::{load_preindex, load_reduced};
mod gen;
struct Item {
polys: Vec<Polygon>,
name: String,
}
impl Item {
fn contains_point(&self, p: &Point) -> bool {
for poly in &self.polys {
if poly.contains_point(*p) {
return true;
}
}
false
}
}
/// Finder works anywhere.
///
/// Finder use a fine tuned Ray casting algorithm implement [geometry-rs]
/// which is Rust port of [geometry] by [Josh Baker].
///
/// [geometry-rs]: https://github.com/ringsaturn/geometry-rs
/// [geometry]: https://github.com/tidwall/geometry
/// [Josh Baker]: https://github.com/tidwall
pub struct Finder {
all: Vec<Item>,
data_version: String,
}
impl Finder {
/// `from_pb` is used when you can use your own timezone data, as long as
/// it's compatible with Proto's desc.
///
/// # Arguments
///
/// * `tzs` - Timezones data.
///
/// # Returns
///
/// * `Finder` - A Finder instance.
#[must_use]
pub fn from_pb(tzs: gen::Timezones) -> Self {
let mut f = Self {
all: vec![],
data_version: tzs.version,
};
for tz in &tzs.timezones {
let mut polys: Vec<Polygon> = vec![];
for pbpoly in &tz.polygons {
let mut exterior: Vec<Point> = vec![];
for pbpoint in &pbpoly.points {
exterior.push(Point {
x: f64::from(pbpoint.lng),
y: f64::from(pbpoint.lat),
});
}
let mut interior: Vec<Vec<Point>> = vec![];
for holepoly in &pbpoly.holes {
let mut holeextr: Vec<Point> = vec![];
for holepoint in &holepoly.points {
holeextr.push(Point {
x: f64::from(holepoint.lng),
y: f64::from(holepoint.lat),
});
}
interior.push(holeextr);
}
let geopoly = geometry_rs::Polygon::new(exterior, interior);
polys.push(geopoly);
}
let item: Item = Item {
name: tz.name.to_string(),
polys,
};
f.all.push(item);
}
f
}
/// Example:
///
/// ```rust
/// use tzf_rs::Finder;
///
/// let finder = Finder::new();
/// assert_eq!("Asia/Shanghai", finder.get_tz_name(116.3883, 39.9289));
/// ```
#[must_use]
pub fn get_tz_name(&self, lng: f64, lat: f64) -> &str {
// let p = &Point::new(lng, lat);
let p = geometry_rs::Point { x: lng, y: lat };
for item in &self.all {
if item.contains_point(&p) {
return &item.name;
}
}
""
}
/// ```rust
/// use tzf_rs::Finder;
/// let finder = Finder::new();
/// println!("{:?}", finder.get_tz_names(116.3883, 39.9289));
/// ```
#[must_use]
pub fn get_tz_names(&self, lng: f64, lat: f64) -> Vec<&str> {
let mut ret: Vec<&str> = vec![];
let p = geometry_rs::Point { x: lng, y: lat };
for item in &self.all {
if item.contains_point(&p) {
ret.push(&item.name);
}
}
ret
}
/// Example:
///
/// ```rust
/// use tzf_rs::Finder;
///
/// let finder = Finder::new();
/// println!("{:?}", finder.timezonenames());
/// ```
#[must_use]
pub fn timezonenames(&self) -> Vec<&str> {
let mut ret: Vec<&str> = vec![];
for item in &self.all {
ret.push(&item.name);
}
ret
}
/// Example:
///
/// ```rust
/// use tzf_rs::Finder;
///
/// let finder = Finder::new();
/// println!("{:?}", finder.data_version());
/// ```
#[must_use]
pub fn data_version(&self) -> &str {
&self.data_version
}
/// Creates a new, empty `Finder`.
///
/// Example:
///
/// ```rust
/// use tzf_rs::Finder;
///
/// let finder = Finder::new();
/// ```
#[must_use]
pub fn new() -> Self {
Self::default()
}
}
/// Creates a new, empty `Finder`.
///
/// Example:
///
/// ```rust
/// use tzf_rs::Finder;
///
/// let finder = Finder::default();
/// ```
impl Default for Finder {
fn default() -> Self {
// let file_bytes = include_bytes!("data/combined-with-oceans.reduce.pb").to_vec();
let file_bytes: Vec<u8> = load_reduced();
Self::from_pb(gen::Timezones::try_from(file_bytes).unwrap_or_default())
}
}
/// deg2num is used to convert longitude, latitude to [Slippy map tilenames]
/// under specific zoom level.
///
/// [Slippy map tilenames]: https://wiki.openstreetmap.org/wiki/Slippy_map_tilenames
///
/// Example:
///
/// ```rust
/// use tzf_rs::deg2num;
/// let ret = deg2num(116.3883, 39.9289, 7);
/// assert_eq!((105, 48), ret);
/// ```
#[must_use]
#[allow(
clippy::cast_precision_loss,
clippy::cast_possible_truncation,
clippy::similar_names
)]
pub fn deg2num(lng: f64, lat: f64, zoom: i64) -> (i64, i64) {
let lat_rad = lat.to_radians();
let n = f64::powf(2.0, zoom as f64);
let xtile = (lng + 180.0) / 360.0 * n;
let ytile = (1.0 - lat_rad.tan().asinh() / PI) / 2.0 * n;
// Possible precision loss here
(xtile as i64, ytile as i64)
}
/// `FuzzyFinder` blazing fast for most places on earth, use a preindex data.
/// Not work for places around borders.
///
/// `FuzzyFinder` store all preindex's tiles data in a `HashMap`,
/// It iterate all zoom levels for input's longitude and latitude to build
/// map key to to check if in map.
///
/// It's is very fast and use about 400ns to check if has preindex.
/// It work for most places on earth and here is a quick loop of preindex data:
/// 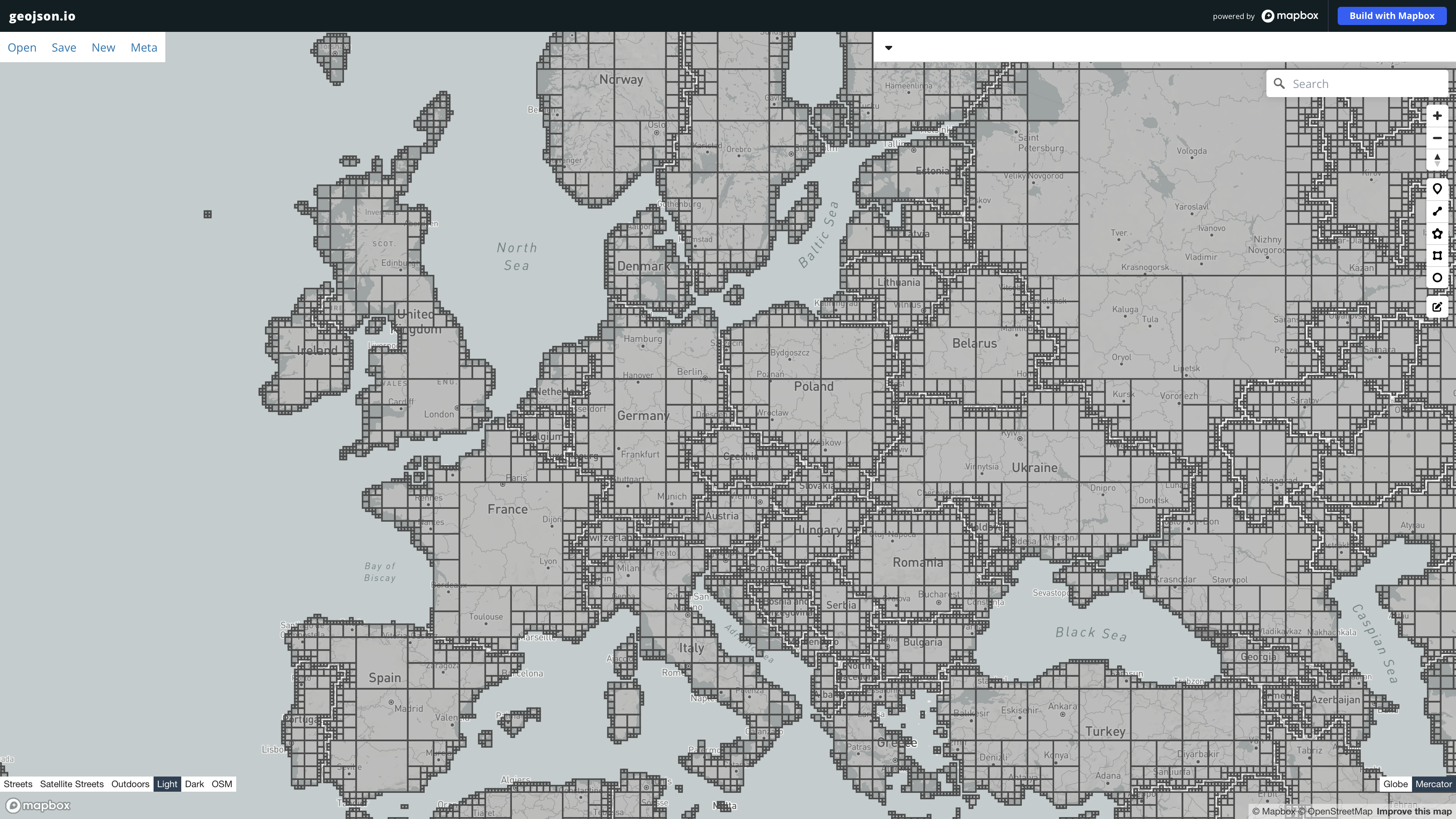
pub struct FuzzyFinder {
min_zoom: i64,
max_zoom: i64,
all: HashMap<(i64, i64, i64), Vec<String>>, // K: <x,y,z>
data_version: String,
}
impl Default for FuzzyFinder {
/// Creates a new, empty `FuzzyFinder`.
///
/// ```rust
/// use tzf_rs::FuzzyFinder;
///
/// let finder = FuzzyFinder::default();
/// ```
fn default() -> Self {
let file_bytes: Vec<u8> = load_preindex();
Self::from_pb(gen::PreindexTimezones::try_from(file_bytes).unwrap_or_default())
}
}
impl FuzzyFinder {
#[must_use]
pub fn from_pb(tzs: gen::PreindexTimezones) -> Self {
let mut f = Self {
min_zoom: i64::from(tzs.agg_zoom),
max_zoom: i64::from(tzs.idx_zoom),
all: HashMap::new(),
data_version: tzs.version,
};
for item in &tzs.keys {
let key = (i64::from(item.x), i64::from(item.y), i64::from(item.z));
f.all.entry(key).or_insert_with(std::vec::Vec::new);
f.all.get_mut(&key).unwrap().push(item.name.to_string());
f.all.get_mut(&key).unwrap().sort();
}
f
}
/// Retrieves the time zone name for the given longitude and latitude.
///
/// # Arguments
///
/// * `lng` - Longitude
/// * `lat` - Latitude
///
/// # Example:
///
/// ```rust
/// use tzf_rs::FuzzyFinder;
///
/// let finder = FuzzyFinder::new();
/// assert_eq!("Asia/Shanghai", finder.get_tz_name(116.3883, 39.9289));
/// ```
///
/// # Panics
///
/// - Panics if `lng` or `lat` is out of range.
/// - Panics if `lng` or `lat` is not a number.
#[must_use]
pub fn get_tz_name(&self, lng: f64, lat: f64) -> &str {
for zoom in self.min_zoom..self.max_zoom {
let idx = deg2num(lng, lat, zoom);
let k = &(idx.0, idx.1, zoom);
let ret = self.all.get(k);
if ret.is_none() {
continue;
}
return ret.unwrap().first().unwrap();
}
""
}
pub fn get_tz_names(&self, lng: f64, lat: f64) -> Vec<&str> {
let mut names: Vec<&str> = vec![];
for zoom in self.min_zoom..self.max_zoom {
let idx = deg2num(lng, lat, zoom);
let k = &(idx.0, idx.1, zoom);
let ret = self.all.get(k);
if ret.is_none() {
continue;
}
for item in ret.unwrap() {
names.push(item);
}
}
names
}
/// Gets the version of the data used by this `FuzzyFinder`.
///
/// # Returns
///
/// The version of the data used by this `FuzzyFinder` as a `&str`.
///
/// # Example:
///
/// ```rust
/// use tzf_rs::FuzzyFinder;
///
/// let finder = FuzzyFinder::new();
/// println!("{:?}", finder.data_version());
/// ```
#[must_use]
pub fn data_version(&self) -> &str {
&self.data_version
}
/// Creates a new, empty `FuzzyFinder`.
///
/// ```rust
/// use tzf_rs::FuzzyFinder;
///
/// let finder = FuzzyFinder::default();
/// ```
#[must_use]
pub fn new() -> Self {
Self::default()
}
}
/// It's most recommend to use, combine both [`Finder`] and [`FuzzyFinder`],
/// if [`FuzzyFinder`] got no data, then use [`Finder`].
pub struct DefaultFinder {
finder: Finder,
fuzzy_finder: FuzzyFinder,
}
impl Default for DefaultFinder {
/// Creates a new, empty `DefaultFinder`.
///
/// # Example
///
/// ```rust
/// use tzf_rs::DefaultFinder;
/// let finder = DefaultFinder::new();
/// ```
fn default() -> Self {
let finder = Finder::default();
let fuzzy_finder = FuzzyFinder::default();
Self {
finder,
fuzzy_finder,
}
}
}
impl DefaultFinder {
/// ```rust
/// use tzf_rs::DefaultFinder;
/// let finder = DefaultFinder::new();
/// assert_eq!("Asia/Shanghai", finder.get_tz_name(116.3883, 39.9289));
/// ```
#[must_use]
pub fn get_tz_name(&self, lng: f64, lat: f64) -> &str {
// The simplified polygon data contains some empty areas where not covered by any timezone.
// It's not a bug but a limitation of the simplified algorithm.
//
// To handle this, auto shift the point a little bit to find the nearest timezone.
for &dx in &[0.0, -0.001, 0.001] {
for &dy in &[0.0, -0.001, 0.001] {
let dlng = dx + lng;
let dlat = dy + lat;
let fuzzy_name = self.fuzzy_finder.get_tz_name(dlng, dlat);
if !fuzzy_name.is_empty() {
return fuzzy_name;
}
let name = self.finder.get_tz_name(dlng, dlat);
if !name.is_empty() {
return name;
}
}
}
""
}
/// ```rust
/// use tzf_rs::DefaultFinder;
/// let finder = DefaultFinder::new();
/// println!("{:?}", finder.get_tz_names(116.3883, 39.9289));
/// ```
#[must_use]
pub fn get_tz_names(&self, lng: f64, lat: f64) -> Vec<&str> {
self.finder.get_tz_names(lng, lat)
}
/// Returns all time zone names as a `Vec<&str>`.
///
/// ```rust
/// use tzf_rs::DefaultFinder;
/// let finder = DefaultFinder::new();
/// println!("{:?}", finder.timezonenames());
/// ```
#[must_use]
pub fn timezonenames(&self) -> Vec<&str> {
self.finder.timezonenames()
}
/// Returns the version of the data used by this `DefaultFinder` as a `&str`.
///
/// Example:
///
/// ```rust
/// use tzf_rs::DefaultFinder;
///
/// let finder = DefaultFinder::new();
/// println!("{:?}", finder.data_version());
/// ```
#[must_use]
pub fn data_version(&self) -> &str {
&self.finder.data_version
}
/// Creates a new instance of `DefaultFinder`.
///
/// ```rust
/// use tzf_rs::DefaultFinder;
/// let finder = DefaultFinder::new();
/// ```
#[must_use]
pub fn new() -> Self {
Self::default()
}
}